@muiの「x-data-grid」でデータ(行)を更新・編集するサンプルコードです。
データのカラムを直接編集することができます。
検証バージョン
- @emotion/react: 11.11.1
- @emotion/styled: 11.11.0
- @mui/material: 5.14.10
- @mui/x-data-grid: 6.15.0
- react: 18.2.0
- react-dom: 18.2.0
Data Gridで表を作成
表を表示するコードのサンプルです、これに更新機能を実装します。
import * as React from 'react';
import Box from '@mui/material/Box';
import { DataGrid, jaJP } from '@mui/x-data-grid';
const dateFormat = (v) => new Date(v.value).toLocaleString('ja-JP', { dateStyle: 'long' });
const columns = [
{ field: 'name', headerName: '名前', width: 180 },
{ field: 'age', headerName: '年齢', type: 'number', width: 80 },
{ field: 'birthday', headerName: '誕生日', type: 'date', width: 200, valueFormatter: dateFormat },
{ field: 'isAdmin', headerName: '管理者', type: 'boolean', width: 80 },
];
const rows = [
{ id: 1, name: '1st', age:10 , birthday: '2023-08-04', isAdmin: false },
{ id: 2, name: '2nd', age:20 , birthday: '2021-02-02', isAdmin: true },
{ id: 3, name: '3rd', age:30 , birthday: '2020-03-03', isAdmin: false },
];
export default function DataGridDemo() {
return (
<Box sx={{ width: '700px' }}>
<DataGrid
columns={columns}
rows={rows}
localeText={jaJP.components.MuiDataGrid.defaultProps.localeText}
autoHeight
/>
</Box>
);
}
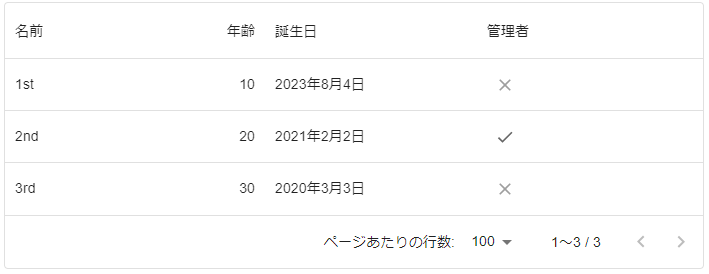
更新機能の実装
更新したい項目に「editable: true」を付けるだけです。
import * as React from 'react';
import Box from '@mui/material/Box';
import Button from '@mui/material/Button';
import { DataGrid, jaJP, useGridApiRef } from '@mui/x-data-grid';
const dateFormat = (v) => new Date(v.value).toLocaleString('ja-JP', { dateStyle: 'long' });
const columns = [
{ field: 'name', headerName: '名前', width: 180, editable: true }, // editable: trueを付ける
{ field: 'age', headerName: '年齢', type: 'number', width: 80, editable: true },
{ field: 'birthday', headerName: '誕生日', type: 'date', width: 200, valueFormatter: dateFormat, editable: true },
{ field: 'isAdmin', headerName: '管理者', type: 'boolean', width: 80, editable: true },
];
const rows = [
{ id: 1, name: '1st', age:10 , birthday: '2023-08-04', isAdmin: false },
{ id: 2, name: '2nd', age:20 , birthday: '2021-02-02', isAdmin: true },
{ id: 3, name: '3rd', age:30 , birthday: '2020-03-03', isAdmin: false },
];
export default function DataGridDemo() {
// データの確認
const apiRef = useGridApiRef();
const checkRows = () => {
apiRef.current.getRowModels().forEach(v => console.log(v));
}
return (
<Box sx={{ width: '700px' }}>
<Box component='div' sx={{ p: 2, textAlign: 'right' }}>
<Button variant="contained" color='primary' onClick={checkRows}>確認</Button>
</Box>
<DataGrid
apiRef={apiRef}
columns={columns}
rows={rows}
localeText={jaJP.components.MuiDataGrid.defaultProps.localeText}
autoHeight
/>
</Box>
);
}
基本的には更新したい項目に「editable: true」を付けるだけで、項目をダブルクリックすれば更新できるようになります。
今回はデータが更新されているか確認するために、現在の状態を確認するためのボタンを付けています。
apiRefを利用してgetRowModels()を呼び出せば、現在のデータをMap型で取得することができます。
ただ、更新するだけなら「editable: true」を付けるだけで完了です。
データ更新前
ダブルクリックで編集モードになります。
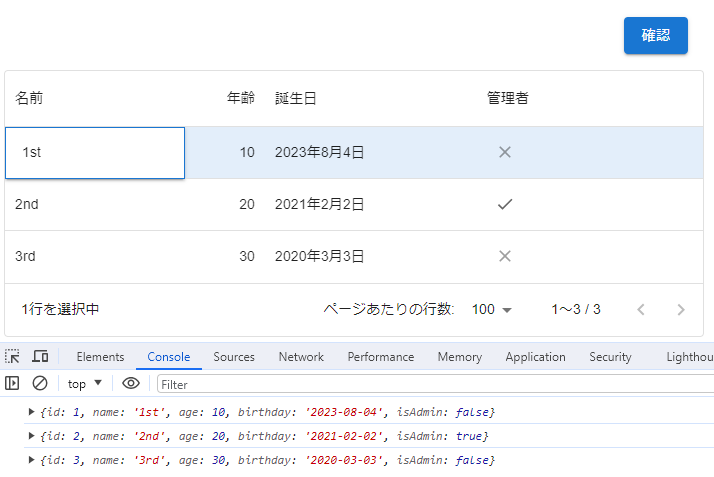
データ更新後
確認ボタンを押すと、consoleに現在のデータが出力されます。
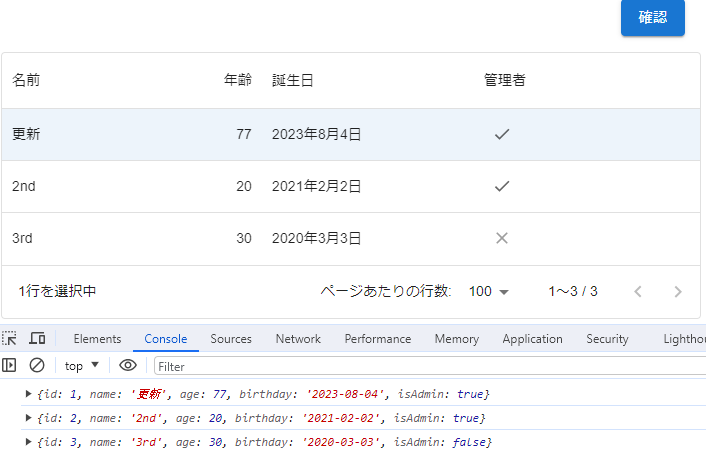
まとめ
@mui/x-data-gridはapiRefが使用できるので、@material-ui/data-gridより楽にコーディングできるようになりました。
有料版の@mui/x-data-grid-proや@mui/x-data-grid-premiumもありますが、ノーマルでもかなり優秀です。
確認ボタンを「保存ボタン」にして押下時にサーバにデータを送れば、サーバに更新・編集後のデータを保存できます。
保存ボタンを押さないとページ遷移したら更新前の状態に戻ります。
reactのアンマウント(unmount)時にデータをサーバに送るようにすれば、ページ遷移時に自動保存とかできると思います。
ただ、これだとリアルタイムでサーバと同期をとることができないですね。
リアルタイムで同期するには、更新・編集イベント発生時にサーバにデータを送る必要があります。
長くなるので、後日別記事にしてアップする予定です。(後日とはいつになるやら・・・)